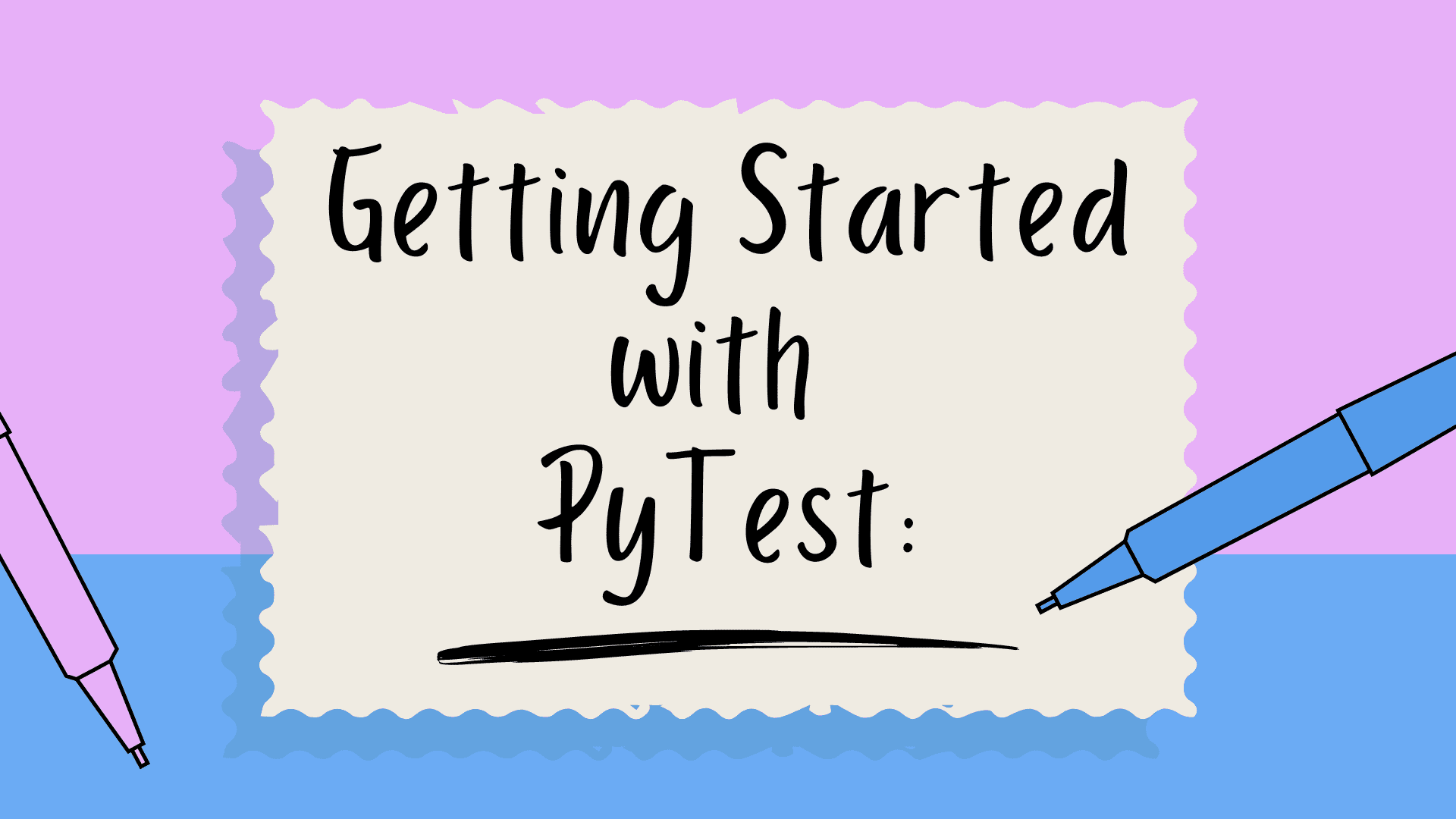
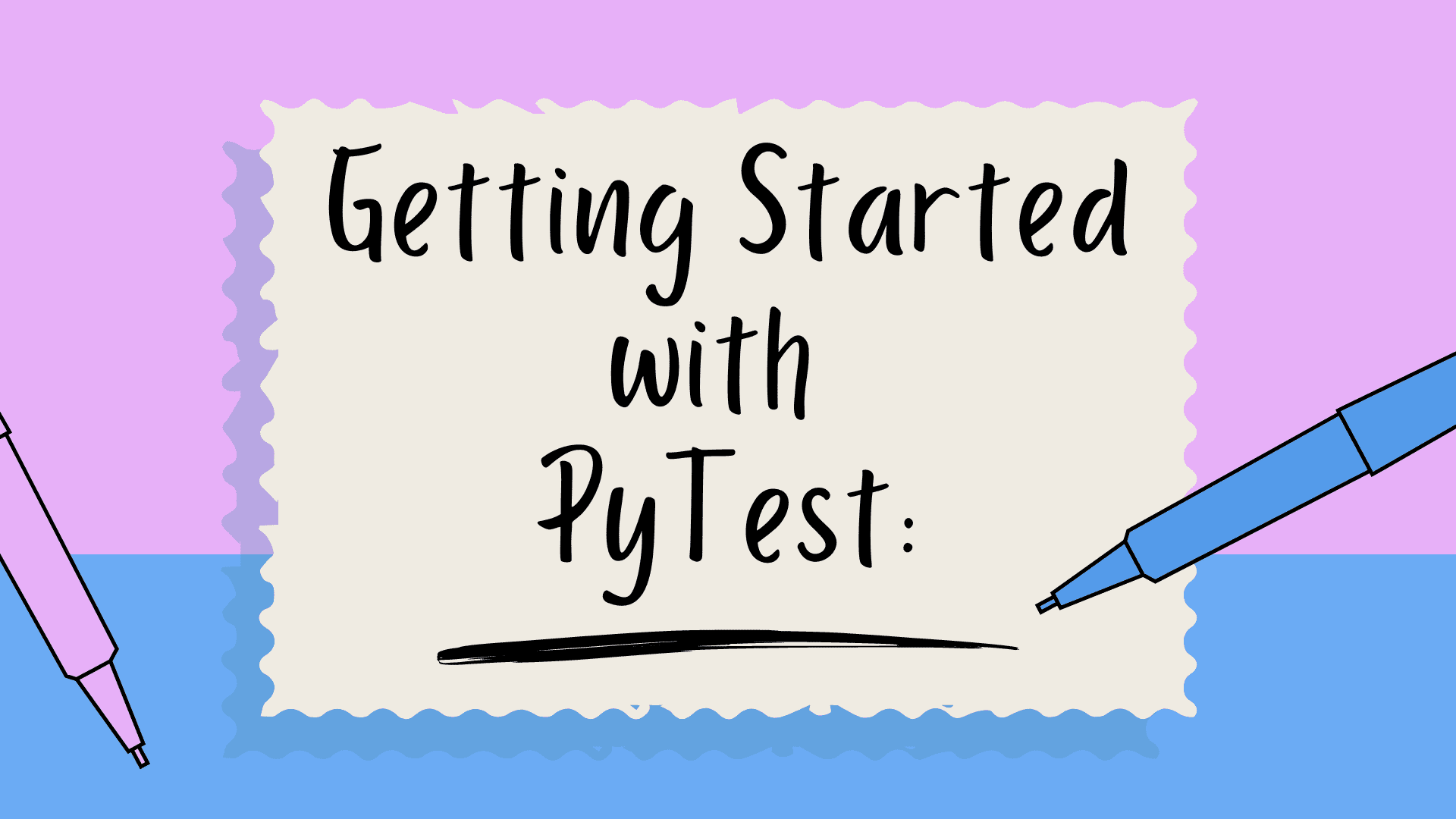
Picture by Creator
Have you ever ever encountered software program that did not work as anticipated? Possibly you clicked a button, and nothing occurred, or a characteristic you have been enthusiastic about turned out to be buggy or incomplete. These points could be irritating for customers and might even result in monetary losses for companies.
To handle these challenges, builders comply with a programming method referred to as test-driven improvement. TDD is all about minimizing software program failures and guaranteeing that the software program meets the meant necessities. These check circumstances describe the anticipated conduct of the code. By writing these checks upfront, builders get a transparent understanding of what they wish to obtain. Check pipelines are a necessary a part of the software program improvement course of for any group. At any time when we make adjustments to our codebase, we have to be certain that they do not introduce new bugs. That is the place check pipelines are available to assist us.
Now, let’s discuss PyTest. PyTest is a Python bundle that simplifies the method of writing and working check circumstances. This full-featured testing device has matured to change into the de facto commonplace for a lot of organizations, because it simply scales for advanced codebases and functionalities.
Advantages of the PyTest Module
- Improved Logging and Check Stories
Upon the execution of checks, we obtain a whole log of all executed checks and the standing of every check case. Within the occasion of failure, a whole stack hint is supplied for every failure, together with the precise values that precipitated an assert assertion to fail. That is extraordinarily helpful for debugging and makes it simpler to hint the precise concern in our code to resolve the bugs. - Automated Discovery of Check Instances
We do not need to manually configure any check case to be executed. All recordsdata are recursively scanned, and all perform names prefixed with “check” are executed routinely. - Fixtures and Parametrization
Throughout check circumstances, particular necessities might not at all times be accessible. For instance, it’s inefficient to fetch a useful resource from the community for testing, and web entry might not be accessible when working a check case. In such situations, if we wish to execute a check that makes web requests, we might want to add stubs that create a dummy response for that particular half. Furthermore, it could be essential to execute a perform a number of instances with completely different arguments to cowl all potential edge circumstances. PyTest makes it easy to implement this utilizing fixtures and parametrization decorators.
Set up
PyTest is offered as a PyPI bundle that may be simply put in utilizing the Python bundle supervisor. To arrange PyTest, it’s good to start out with a contemporary atmosphere. To create a brand new Python digital atmosphere, use the beneath instructions:
python3 -m venv venv
supply venv/bin/activate
To arrange the PyTest module, you’ll be able to set up the official PyPI bundle utilizing pip:
Working your First Check Case
Let’s dive into writing and working your very first check case in Python utilizing PyTest. We’ll begin from scratch and construct a easy check to get a really feel for the way it works.
Structuring a Python Challenge
Earlier than we begin writing checks, it is important to arrange our undertaking correctly. This helps hold issues tidy and manageable, particularly as our tasks develop. We’ll comply with a standard apply of separating our utility code from our check code.
This is how we’ll construction our undertaking:
pytest_demo/
│
├── src/
│ ├── __init__.py
│ ├── sorting.py
│
├── checks/
│ ├── __init__.py
│ ├── test_sorting.py
│
├── venv/
Our root listing pytest_demo accommodates separate src and checks directories. Our utility code resides in src, whereas our check code lives in checks.
Writing a Easy Program and Its Related Check Case
Now, let’s create a primary sorting program utilizing the bubble type algorithm. We’ll place this in src/sorting.py:
# src/sorting.py
def bubble_sort(arr):
for n in vary(len(arr)-1, 0, -1):
for i in vary(n):
if arr[i] > arr[i + 1]:
arr[i], arr[i + 1] = arr[i + 1], arr[i]
return arr
We have applied a primary Bubble Kind algorithm, a easy but efficient technique to type parts in an inventory by repeatedly swapping adjoining parts if they’re within the unsuitable order.
Now, let’s guarantee our implementation works by writing complete check circumstances.
# checks/test_sorting.py
import pytest
from src.sorting import bubble_sort
def test_always_passes():
assert True
def test_always_fails():
assert False
def test_sorting():
assert bubble_sort([2,3,1,6,4,5,9,8,7]) == [1,2,3,4,5,6,7,8,9]
In our check file, we have written three completely different check circumstances. Observe how every perform identify begins with the check prefix, which is a rule PyTest follows to acknowledge check features.
We import the bubble type implementation from the supply code within the check file. This may now be utilized in our check circumstances. Every check should have an “assert” assertion to test if it really works as anticipated. We give the sorting perform an inventory that is not so as and evaluate its output with what we anticipate. In the event that they match, the check passes; in any other case, it fails.
As well as, We have additionally included two easy checks, one which at all times passes and one other that at all times fails. These are simply placeholder features which can be helpful for checking if our testing setup is working appropriately.
Executing Checks and Understanding the Output
We are able to now run our checks from the command line. Navigate to your undertaking root listing and run:
It will recursively search all recordsdata within the checks listing. All features and courses that begin with the check prefix can be routinely acknowledged as a check case. From our checks listing, it would search within the test_sorting.py file and run all three check features.
After working the checks, you’ll see an output much like this:
===================================================================
check session begins ====================================================================
platform darwin -- Python 3.11.4, pytest-8.1.1, pluggy-1.5.0
rootdir: /pytest_demo/
collected 3 objects
checks/test_sorting.py .F. [100%]
========================================================================= FAILURES
=========================================================================
____________________________________________________________________ test_always_fails _____________________________________________________________________
def test_always_fails():
> assert False
E assert False
checks/test_sorting.py:22: AssertionError
================================================================= brief check abstract information ==================================================================
FAILED checks/test_sorting.py::test_always_fails - assert False
===============================================================
1 failed, 2 handed in 0.02s ================================================================
When working the PyTest command line utility, it shows the platform metadata and the entire check circumstances that can be run. In our instance, three check circumstances have been added from the test_sorting.py file. Check circumstances are executed sequentially. A dot (“.”) represents that the check case handed whereas an “F” represents a failed check case.
If a check case fails, PyTest offers a traceback, which reveals the particular line of code and the arguments that precipitated the error. As soon as all of the check circumstances have been executed, PyTest presents a remaining report. This report consists of the entire execution time and the variety of check circumstances that handed and failed. This abstract provides you a transparent overview of the check outcomes.
Operate Parametrization for A number of Check Instances
In our instance, we check just one situation for the sorting algorithm. Is that enough? Clearly not! We have to check the perform with a number of examples and edge circumstances to make sure there aren’t any bugs in our code.
PyTest makes this course of simple for us. We use the parametrization decorator supplied by PyTest so as to add a number of check circumstances for a single perform. The code seems as follows:
@pytest.mark.parametrize(
"input_list, expected_output",
[
([], []),
([1], [1]),
([53,351,23,12], [12,23,53,351]),
([-4,-6,1,0,-2], [-6,-4,-2,0,1])
]
)
def test_sorting(input_list, expected_output):
assert bubble_sort(input_list) == expected_output
Within the up to date code, we’ve got modified the test_sorting perform utilizing the pytest.mark.parametrize decorator. This decorator permits us to move a number of units of enter values to the check perform. The decorator expects two parameters: a string representing the comma-separated names of the perform parameters, and an inventory of tuples the place every tuple accommodates the enter values for a selected check case.
Observe that the perform parameters have the identical names because the string handed to the decorator. This can be a strict requirement to make sure the right mapping of enter values. If the names do not match, an error can be raised throughout check case assortment.
With this implementation, the test_sorting perform can be executed 4 instances, as soon as for every set of enter values specified within the decorator. Now, let’s check out the output of the check circumstances:
===================================================================
check session begins
====================================================================
platform darwin -- Python 3.11.4, pytest-8.1.1, pluggy-1.5.0
rootdir: /pytest_demo
collected 6 objects
checks/test_sorting.py .F.... [100%]
=======================================================================
FAILURES ========================================================================
____________________________________________________________________ test_always_fails _____________________________________________________________________
def test_always_fails():
> assert False
E assert False
checks/test_sorting.py:11: AssertionError
=================================================================
brief check abstract information ==================================================================
FAILED checks/test_sorting.py::test_always_fails - assert False
===============================================================
1 failed, 5 handed in 0.03s ================================================================
On this run, a complete of six check circumstances have been executed, together with 4 from the test_sorting perform and two dummy features. As anticipated, solely the dummy check case failed.
We are able to now confidently say that our sorting implementation is right 🙂
Enjoyable Apply Process
On this article, we’ve got launched the PyTest module and demonstrated its utilization by testing a bubble type implementation with a number of check circumstances. We coated the essential performance of writing and executing check circumstances utilizing the command line utility. This ought to be sufficient to get you began with implementing testing on your personal code bases. To make your understanding of PyTest higher, this is a enjoyable apply activity for you:
Implement a perform referred to as validate_password that takes a password as enter and checks if it meets the next standards:
- Incorporates a minimum of 8 characters
- Incorporates a minimum of one uppercase letter
- Incorporates a minimum of one lowercase letter
- Incorporates a minimum of one digit
- Incorporates a minimum of one particular character (e.g., !, @, #, $, %)
Write PyTest check circumstances to validate the correctness of your implementation, overlaying varied edge circumstances. Good Luck!
Kanwal Mehreen Kanwal is a machine studying engineer and a technical author with a profound ardour for information science and the intersection of AI with medication. She co-authored the e book “Maximizing Productiveness with ChatGPT”. As a Google Technology Scholar 2022 for APAC, she champions variety and educational excellence. She’s additionally acknowledged as a Teradata Range in Tech Scholar, Mitacs Globalink Analysis Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having based FEMCodes to empower ladies in STEM fields.