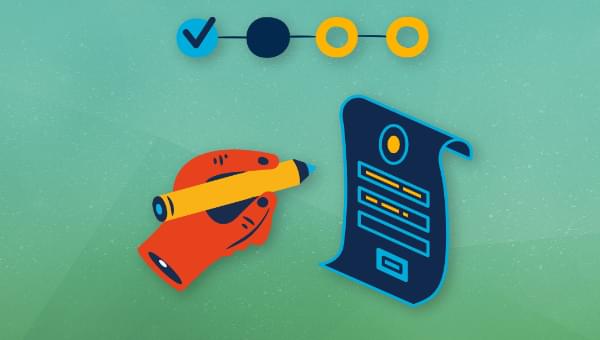
On this article, we’ll speak about React kind builders, try their core options, take a look at some necessary points to think about when choosing a kind builder, and discover some in style choices.
These in style choices embody:
Kinds play a important position in participating customers, gathering important data, enabling necessary options in numerous purposes, and permitting customers to enter data.
Nonetheless, constructing and controlling numerous sorts of types from scratch can take time and an entire lot of effort. And that’s the place kind builders are available.
Type builders are made to simplify kind creation in React purposes, making the person expertise easy and pleasant.
Understanding React Type Builders
React kind builders make it straightforward to create and handle types in React apps. Among the many options they provide are:
- inputs, drop-downs, and extra are constructed into pre-built types
- administration of kind states
- the validation course of
- submission of kind knowledge
Type builders provide numerous advantages, resembling:
- making the event course of quicker as a result of there’s much less code writing and state administration
- providing glossy interactions, clear validation, and user-friendly design
- dealing with on a regular basis duties resembling validation
- providing accessibility options to make types extra usable
Type builders provide numerous options to builders, resembling:
- deciding on a builder and following its integration information
- integrating the shape based mostly on the builder’s interface (both drag-and-drop or code-based)
- creating components and defining validation guidelines, labels, and knowledge varieties
- describe how the shape reacts to person interactions
The assorted options kind builders would possibly provide embody:
- drag-and-drop, which is accessible for non-technical customers
- code customization
- a mix of code modifying and drag-and-drop performance
SurveyJS
SurveyJS Form Builder is an open-source UI part in React that completely blends with any backend system and provides the chance to create and magnificence many dynamic HTML types in a React software.
You’ll be able to simply expertise all of the options of this manner builder by way of this fast demo with none required integration.
SurveyJS options
- a devoted GUI for conditional guidelines
- kind branching and an built-in CSS theme editor for customized kind styling and branding
- TypeScript assist
- integration with any backend framework (examples for PHP, Node.js, and ASP.NET included)
- producing JSON kind definitions (schemas) in actual time
- a no-code, drag-and-drop interface that makes kind creation accessible to anybody
Within the sections under, we’ll cowl the step-by-step strategies to get began with the SurveyJS kind builder part in a React software.
SurveyJS set up
Set up the survey-creator-react
npm package. The very first thing is to put in the survey-creator-react (rendering code) npm package deal utilizing the command under:
npm set up survey-creator-react --save
The command above makes positive the survey-creator-core
package deal is put in routinely as a dependency.
SurveyJS kinds configuration
The following step is to import the Survey Creator and SurveyJS Type Library stylesheets as indicated under:
import "survey-core/defaultV2.min.css";
import "survey-creator-core/survey-creator-core.min.css";
Survey creator configuration
The following step is to configure the Survey Creator part. The code under is used to configure the Survey Creator part by specifying its properties in a configuration object:
const creatorOptions = {
showLogicTab: true,
isAutoSave: true
};
The thing above permits the next properties:
showLogicTab
shows the Logic tab within the tab panelisAutoSave
routinely saves the survey JSON schema on each change
Now, we have to cross the configuration object to the SurveyCreator
constructor, as proven within the code under, to instantiate Survey Creator after which assign the produced occasion to a relentless that will probably be used later to render the part:
import { SurveyCreator } from "survey-creator-react";
export perform SurveyCreatorWidget() {
const creator = new SurveyCreator(creatorOptions);
}
Rendering Survey Creator
To render Survey Creator, all that’s wanted is to import the SurveyCreatorComponent
, embody it within the template, and cross the occasion we created within the earlier step to the part’s creator
attribute:
import { SurveyCreatorComponent, SurveyCreator } from "survey-creator-react";
export perform SurveyCreatorWidget() {
const creator = new SurveyCreator(creatorOptions);
return (
<SurveyCreatorComponent creator={creator} />
)
}
Saving and loading survey mannequin schemas
By default, Survey Creator makes survey mannequin schemas as JSON objects, which makes it straightforward to persist the objects on the server, save updates and restore earlier saved schemas.
All that’s wanted to avoid wasting a JSON object is to implement the saveSurveyFunc
perform, which accepts two arguments:
saveNo
. That is an incremental quantity of the present change.callback
. It is a callback perform. When referred to as, thesaveNo
should be handed as the primary argument whereas the second argument is about totrue
orfalse
based mostly on whether or not the server utilized or rejected the change.
The code under reveals use the saveSurveyFunc
perform to avoid wasting a survey mannequin schema in a neighborhood storage or an online service:
export perform SurveyCreatorWidget() {
creator.saveSurveyFunc = (saveNo, callback) => {
window.localStorage.setItem("survey-json", creator.textual content);
callback(saveNo, true);
saveSurveyJson(
"https://your-web-service.com/",
creator.JSON,
saveNo,
callback
);
};
}
perform saveSurveyJson(url, json, saveNo, callback) {
}
Extra particulars on load a survey mannequin schema JSON into Survey Creator and examine a survey JSON schema earlier than saving it when operating a Node.js server might be discovered here.
Managing picture uploads in SurveyJS
Including a logo or background to a survey is a standard requirement. We are able to both add them within the survey header or inside Image and Image Picker questions when making a survey, and SurveyJS has made this straightforward to do. All that’s wanted is to embed them within the survey and theme JSON schemas as Base64 URLs.
Nonetheless, this technique will increase the schema measurement. One good method to get round that is to add photographs to a server and save solely picture hyperlinks within the JSON schemas.
The code under reveals use the onUploadFile
occasion. The choices.recordsdata
parameter saves the photographs despatched to the server:
export perform SurveyCreatorWidget() {
creator.onUploadFile.add((_, choices) => {
const formData = new FormData();
choices.recordsdata.forEach(file => {
formData.append(file.identify, file);
});
fetch("https://instance.com/uploadFiles", {
technique: "submit",
physique: formData
}).then(response => response.json())
.then(end result => {
choices.callback(
"success",
"https://instance.com/recordsdata?identify=" + end result[options.files[0].identify]
);
})
.catch(error => {
choices.callback('error');
});
});
}
The onUploadFile
occasion is used to implement picture add. Its choices.recordsdata
parameter shops the photographs we should always ship to our server. When the server returns a picture hyperlink, the choices.callback(standing, imageLink)
technique is named with a success
because the standing
parameter handed and a hyperlink to the uploaded picture because the imageLink
parameter.
Now, to view the applying, we have to run npm run begin
within the command line and open http://localhost:3000/
within the browser.
Different helpful SurveyJS hyperlinks
You might also discover these SurveyJS sources helpful:
The survey-creator supply code is publicly accessible right here on GitHub.
FormBuilder
FormBuilder is a drag-and-drop React kind builder library for creating internet types with a easy however highly effective internet interface.
Right here’s a fast demo to expertise all of the options of the FormBuilder with none required integration.
The next npm packages make up FormBuilder:
FormBuilder options
- an online interface with drag and drop performance
- adaptive format
- kind validation
- built-in internet parts based mostly on React Suite library
- straightforward integration of customized parts
- export kind to JSON and import kind from JSON
- highly effective internationalization
- customized actions
- computable properties
- templates (types inside a kind)
Making a easy kind demo with FormBuilder
To create a easy demo kind, we’ll observe the directions within the Getting Started part of the FormBuilder docs.
Following the information and opening the demo web page within the browser, we’ll see the shape builder editor web page much like the picture under.
We construct the types right here, as seen within the directions information. For the sake of this demo, we’ve created a mini-application kind, added an onClick
occasion handler for the shape, and added validation on the enter area. We’ve additionally added a tooltip for the button and altered the format of the error message show.
In contrast to SurveyJS — which permits even non-techies to arrange all kind configurations, together with their conduct, with out a line of code — FormBuilder requires manually including code for actions, as proven within the picture under.
Including the FormViewer part
Now, we are able to add the FormViewer part, liable for displaying the shape in FormBuilder.
The FormBuilder makes use of the FormViewer part to show the shape within the heart panel.
However we have to set up the package deal first by utilizing this command:
npm set up @react-kind-builder/core @react-kind-builder/parts-rsuite
Now, we are able to use the FormViewer part with components from the React Suite library to render the Software kind by pasting the code under within the App.js file:
import React from 'react'
import {view} from '@react-form-builder/components-rsuite'
import {FormViewer} from '@react-form-builder/core'
const kind = `{
"kind": {
"key": "Display",
"kind": "Display",
"props": {},
"kids": [
{
"key": "RsInput 1",
"type": "RsInput",
"props": {}
}
]
}
}`
perform App() {
return <FormViewer view={view} getForm={_ => kind}/>
}
export default App
View a fuller instance
import { FormViewer, IFormViewer } from '@react-form-builder/core'
import { useEffect, useRef } from 'react'
import { view } from '@react-form-builder/components-rsuite'
const FormJSON = `
{
"model": "1",
"actions": {
"logValue": {
"physique": "console.log('FirstName', e.knowledge.identify, 'LastName', e.knowledge.lastname)",
"params": {}
}
},
"tooltipType": "RsTooltip",
"errorType": "RsErrorMessage",
"kind": {
"key": "Display",
"kind": "Display",
"props": {},
"kids": [
{
"key": "RsHeader 1",
"type": "RsHeader",
"props": {
"content": {
"value": "Application Form"
}
},
"css": {
"any": {
"object": {
"textAlign": "center"
}
}
}
},
{
"key": "name",
"type": "RsInput",
"props": {
"label": {
"value": "First Name"
},
"placeholder": {
"value": "First Name"
}
},
"schema": {
"validations": [
{
"key": "required"
},
{
"key": "min",
"args": {
"limit": 3
}
}
]
},
"occasions": {
"onChange": [
{
"name": "validate",
"type": "common"
},
{
"name": "logValue",
"type": "code"
}
]
}
},
{
"key": "lastname",
"kind": "RsInput",
"props": {
"label": {
"worth": "Final Identify"
},
"placeholder": {
"worth": "Final Identify"
}
},
"occasions": {
"onChange": [
{
"name": "validate",
"type": "common"
},
{
"name": "logValue",
"type": "code"
}
]
},
"schema": {
"validations": [
{
"key": "required"
},
{
"key": "min",
"args": {
"limit": 3
}
}
]
}
},
{
"key": "RsButton 1",
"kind": "RsButton",
"props": {
"kids": {
"worth": "Submit"
}
},
"occasions": {
"onClick": [
{
"name": "validate",
"type": "common"
},
{
"name": "logValue",
"type": "code"
}
]
}
}
]
},
"localization": {},
"languages": [
{
"code": "en",
"dialect": "US",
"name": "English",
"description": "American English",
"bidi": "ltr"
}
],
"defaultLanguage": "en-US"
}
`
const formName = 'Instance'
async perform getFormFn(identify?: string) {
if (identify === formName) return FormJSON
throw new Error(`Type '${identify}' isn't discovered.`)
}
perform App() {
const ref = useRef<IFormViewer>(null)
useEffect(() => {
if (ref.present) {
console.log('Viewer', ref.present)
}
}, [])
return (
<FormViewer
view={view}
getForm={getFormFn}
formName={formName}
initialData={({})}
viewerRef={ref}
/>
)
}
export default App
Subsequent is to verify the shape by visiting localhost:3000
within the browser to view the applying kind.
Beneath is an instance of what we should always see on the display.
Tripetto
Tripetto is a kind device that gives an entire and distinctive answer for creating and operating types and surveys.
Tripetto comes as a SaaS application, precisely like Typeform and SurveyMonkey.
Tripetto’s operating operation consists of three core pillars:
- a visible kind builder to create types (kind designer)
- runners to run these types (and gather responses)
- blocks (query varieties) to be used within the types
One benefit of Tripetto is that the operating pillars above can be found as totally client-side parts.
They run within the context of the person’s browser and don’t depend on a selected backend, which suggests they’ll serve in any client-side setting that helps JavaScript.
Additionally, it provides complete freedom in dealing with the info that Tripetto generates or consumes.
The backend may also be no matter we would like.
Tripetto options
How Tripetto works
There are numerous choices for utilizing Tripetto’s visible kind builder to create types. We are able to combine it into any mission, however it’s elective. The only option for any mission is determined by among the required wants.
Among the many choices out there are:
However we’ll use the Tripetto Studio internet app to create a kind and clarify use the Tripetto runner to run it.
The Tripetto Studio internet app permits anybody who wants a kind or survey. Whether or not they want a kind for an internet site or need to create a survey that may be shared utilizing a direct hyperlink, it’s all potential with the Studio.
Making a easy demo kind with Tripetto
To create a easy kind, all we have to do is go to tripetto.app and begin constructing our kind.
Right here, we’ve made an software kind with out creating an account following the documentation guide.
But when we need to save (and publish) our kind and retailer it, we have to create an account by clicking on the person icon within the high proper nook of the applying.
Now that we’ve constructed our kind, we’ll run it and prolong the shape to incorporate extra query varieties. Right here’s extra details about doing that within the documentation.
Type.io
Form.io permits the event of form-based progressive internet purposes. It permits builders to create types speedily utilizing a painless drag-and-drop kind builder interface. Creating these types generates a JSON schema to render the types inside the progressive software dynamically and routinely create the API to obtain the info when the shape is submitted.
Type.io supplies every little thing wanted to construct enterprise course of workflow purposes with little effort and sophisticated, form-driven purposes inside a number of occasions with out compromising safety or sanity.
Type.io options
The next are among the important options of Type.io:
- OAuth suppliers
- dynamic types
- straightforward function enhancement
- person administration and auth
- types and knowledge administration
- role-based entry management
- computerized API creation
- a drag-and-drop interface
- superior conditional logic
The Type.io web site accommodates extra details about its features.
Getting began with Type.io
To get began with Type.io, listed below are the essential steps you may take:
Following the described steps above, we’ll find yourself with one thing like the shape pictured under.
We are able to do many different issues with the FormView software, resembling white labeling, altering the emblem, navigating to a thankyou web page, and so forth. For extra details about the FormView software, please try the FormView user guide.
A Comparability Between SurveyJS and FormBuilder
Options | SurveyJS | FormBuilder |
---|---|---|
Drag and drop kind builder | Sure | Sure, however requires handbook coding for actions |
JSON types | Sure | Sure |
Customizable format | Sure | Sure |
Ease of Use | Sure (nice documentation) | Sure |
Language translation | Sure | Sure |
Conditional logic | Sure | Sure |
Pricing | Free with important options | Free with restricted options |
Please seek advice from the documentation for added options out there in SurveyJS and FormBuilder.
Selecting the Proper Type Builder
When selecting a kind builder device for a company or mission, we clearly want to make sure that the device we select has all the suitable options to satisfy our necessities.
Listed below are some customary options to search for when deciding on the precise kind builder software program for a enterprise or mission:
- user-friendliness
- the power to customise types simply
- conditional logic and a variety of query varieties
- straightforward integration with third-party programs
- automation of labor processes
- free trial and fundamental demo choices
- an offline options device
- reporting and analytics
Conclusion
Kinds are useful in nearly each enterprise and trade to gather buyer data and suggestions. A robust kind builder device helps us create skilled trying types for this goal. On this article, we’ve launched 4 highly effective React kind builder instruments and given a quick overview of how they work. Optimistically, at the very least certainly one of them is perhaps what you want.
Different choices you would possibly like to take a look at embody Amplify Studio’s Form Builder and the BEEKAI kind builder.
To study extra about working with types in React, try Working with Forms in React.