At the moment we’ll check out the right way to create a fantastic animation in React Three Fiber. The concept is to indicate a 3D scene within the background that appears like a distorted glassy view of a textual content ring, continuously rotating round its personal axis. It’s a fantastic design component that may very well be used as an animated background. The animation is just not very advanced, excellent for these serious about getting began with 3D animation and extra.
It is a React particular tutorial so you’ll want to have an preliminary React app arrange in order that we are able to begin.
First we might want to set up React Three Fiber:
npm set up three @react-three/fiber
After that we are going to put together the canvas for rendering our 3D scene.
We first import the canvas from @react-three/fiber
, type the primary component to be 100% viewport width and top and use the imported Canvas part. We’ll additionally type it to have a black background.
import { Canvas } from "@react-three/fiber";
export default perform App() {
return (
<important className="h-screen w-screen">
<Canvas className="bg-black">
</Canvas>
</important>
);
}
The following step is to organize our 3D part that will likely be rendered contained in the Canvas
which we’ll name ring as a result of we’ll be wrapping the textual content within the type of a hoop. The way in which we are able to think about that is as if we took our textual content and wrapped it round a bottle which might be forming this so known as “TextRing”.
interface Props {
radius: quantity;
top: quantity;
segments: quantity;
}
export default perform Ring({ radius, top, segments }: Props) {
return (
<mesh>
<cylinderGeometry args={[radius, radius, height, segments]} />
<meshBasicMaterial />
</mesh>
);
}
After you have got written the part the one remaining factor left to do is add it to the primary scene in our Canvas:
import { Canvas } from "@react-three/fiber";
import Ring from "./ring";
export default perform Scene() {
return (
<Canvas className="bg-black">
<Ring radius={2} top={4} segments={32} />
</Canvas>
);
}
After this you have to be seeing this in your display:
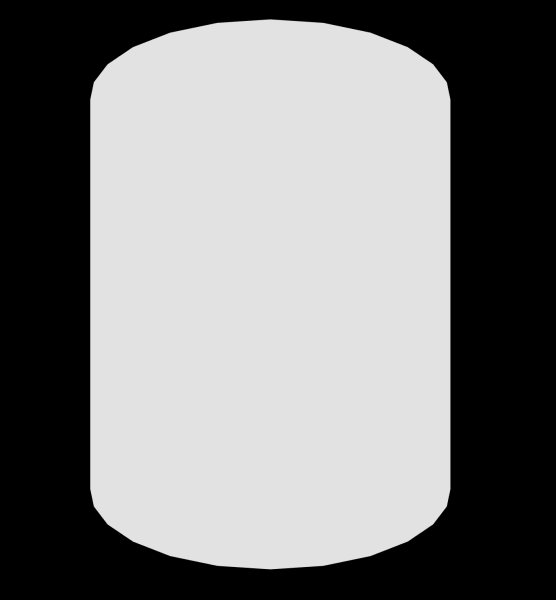
Within the subsequent step we must embody our textual content within the scene and with a view to to that we are going to set up one other bundle known as @react-three/drei
that has a a number of superior issues. On this case we use the Textual content part.
npm set up @react-three/drei
After we put in our bundle we have now to regulate our part with a view to present our textual content and wrap it round our cylinder:
import { Textual content } from "@react-three/drei";
interface Props {
textual content: string;
radius: quantity;
top: quantity;
segments: quantity;
}
export default perform Ring({ textual content, radius, top, segments }: Props) {
// Calculate positions for textual content
const textPositions: { x: quantity; z: quantity }[] = [];
const angleStep = (2 * Math.PI) / textual content.size;
for (let i = 0; i < textual content.size; i++) {
const angle = i * angleStep;
const x = radius * Math.cos(angle);
const z = radius * Math.sin(angle);
textPositions.push({ x, z });
}
return (
<group>
<mesh>
<cylinderGeometry args={[radius, radius, height, segments]} />
<meshBasicMaterial />
</mesh>
{textual content.cut up("").map((char: string, index: quantity) => (
<Textual content
key={index}
place={[textPositions[index].x, 0, textPositions[index].z]}
rotation={[0, -angleStep * index + Math.PI / 2, 0]}
fontSize={0.3}
lineHeight={1}
letterSpacing={0.02}
coloration="white"
textAlign="heart"
>
{char}
</Textual content>
))}
</group>
);
}
So, there may be plenty of issues happening right here, however to clarify it in fundamental terminology, we take every character and more and more place it round in a round place. We use the radius that we used for the cylinder to place all of the letters.
We move any textual content into the Ring part after this, after which will probably be neatly wrapper across the cylinder like this:
export default perform Scene() {
return (
<Canvas className="bg-black">
<Ring
radius={2}
top={4}
segments={32}
textual content="X X X X X X X X X X X X X X X X X X X X X X X X X X X X "
/>
</Canvas>
);
}
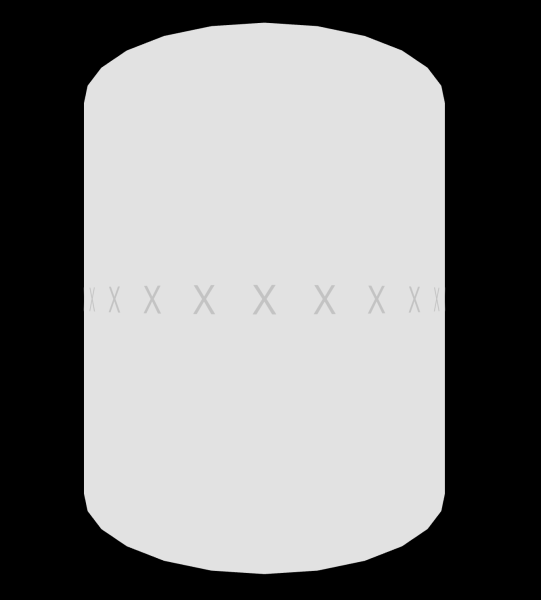
To start out animating issues, we’ll merely take the group and rotate it alongside its x,y,z axis. For that we are going to use the useFrame
hook from R3F and we’ll entry the component through the useRef
that we are going to hyperlink to the group component.
import { useRef } from "react";
import { useFrame } from "@react-three/fiber";
import { Textual content } from "@react-three/drei";
interface Props {
textual content: string;
radius: quantity;
top: quantity;
segments: quantity;
}
export default perform Ring({ textual content, radius, top, segments }: Props) {
const ref = useRef<any>();
// Rotate the textual content
useFrame(() => {
ref.present.rotation.y += 0.01;
ref.present.rotation.x += 0.01;
ref.present.rotation.z += 0.01;
});
// Calculate positions for textual content
const textPositions: { x: quantity; z: quantity }[] = [];
const angleStep = (2 * Math.PI) / textual content.size;
for (let i = 0; i < textual content.size; i++) {
const angle = i * angleStep;
const x = radius * Math.cos(angle);
const z = radius * Math.sin(angle);
textPositions.push({ x, z });
}
return (
<group ref={ref}>
<mesh>
<cylinderGeometry args={[radius, radius, height, segments]} />
<meshBasicMaterial />
</mesh>
{textual content.cut up("").map((char: string, index: quantity) => (
<Textual content
key={index}
place={[textPositions[index].x, 0, textPositions[index].z]}
rotation={[0, -angleStep * index + Math.PI / 2, 0]}
fontSize={0.3}
lineHeight={1}
letterSpacing={0.02}
coloration="white"
textAlign="heart"
>
{char}
</Textual content>
))}
</group>
);
}
The one remaining step left to do is to barely distort the component a bit and make it glass-like trying. Fortunately, drei has a cloth that helps us with that known as MeshTransmissionMaterial
:
import { useRef } from "react";
import { useFrame } from "@react-three/fiber";
import { MeshTransmissionMaterial, Textual content } from "@react-three/drei";
interface Props {
textual content: string;
radius: quantity;
top: quantity;
segments: quantity;
}
export default perform Ring({ textual content, radius, top, segments }: Props) {
const ref = useRef<any>();
// Rotate the textual content
useFrame(() => {
ref.present.rotation.y += 0.01;
ref.present.rotation.x += 0.01;
ref.present.rotation.z += 0.01;
});
// Calculate positions for textual content
const textPositions: { x: quantity; z: quantity }[] = [];
const angleStep = (2 * Math.PI) / textual content.size;
for (let i = 0; i < textual content.size; i++) {
const angle = i * angleStep;
const x = radius * Math.cos(angle);
const z = radius * Math.sin(angle);
textPositions.push({ x, z });
}
return (
<group ref={ref}>
<mesh>
<cylinderGeometry args={[radius, radius, height, segments]} />
<MeshTransmissionMaterial
bottom
backsideThickness={5}
thickness={2}
/>
</mesh>
{textual content.cut up("").map((char: string, index: quantity) => (
<Textual content
key={index}
place={[textPositions[index].x, 0, textPositions[index].z]}
rotation={[0, -angleStep * index + Math.PI / 2, 0]}
fontSize={0.3}
lineHeight={1}
letterSpacing={0.02}
coloration="white"
textAlign="heart"
>
{char}
</Textual content>
))}
</group>
);
}
And with that we have now our stunning rotating glassy textual content ring:
I hope you loved this tutorial!
I’m open for collaborations and tasks, so drop me a line in case you’d wish to work with me!