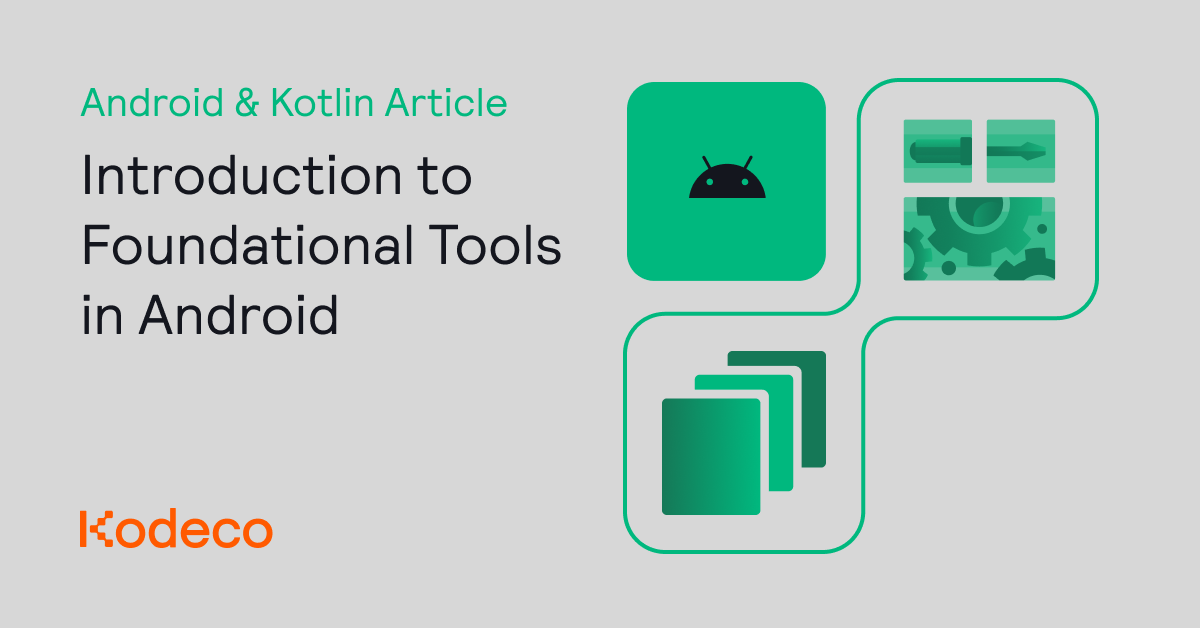
Each job requires instruments, whether or not constructing a brand new bookshelf or creating a brand new Android app. These instruments are crucial to the duty at hand. Fortunately, with Android growth, you don’t need to spend your life financial savings to accumulate them. All these instruments are free!
Android Studio, the Kotlin Programming Language, and Jetpack Compose are the three most important instruments you’ll use in your Android profession. After all, you should utilize many different instruments to construct your apps, however you’ll use these in each app.
Android Studio is an built-in developer surroundings that permits you to write code, create emulators, and debug code because it runs. Kotlin is a contemporary object-oriented programming language that’s simple to jot down and perceive. Jetpack Compose is a person interface toolkit that helps you lay out the assorted parts of your apps, corresponding to pictures and buttons.
On this article, you’ll dip your toe in Jetpack Compose. If you wish to discover all these matters in depth, join Kodeco’s Introduction to Foundational Tools in Android program. This program walks you thru each step of the setup course of, and also you’ll even create a small app.
Create a Easy Android App
On this module, Fuad Kamal teaches you easy methods to create a easy chat app in Jetpack Compose. Within the course of, you’ll:
Study composables. Composables are the constructing blocks of your person interface. You’ll discover ways to outline a composable and join them like Lego bricks to design a killer person interface.
Perceive some person interface parts used to construct a UI. You’ll be taught to make use of buttons, rows, textual content, and different parts in your Jetpack Compose app.
Mix composables right into a singular composable. You’ll discover ways to create customized composables that you could share all through your app and even with others.
Seeing it in Motion
The default Exercise created for you comprises the next operate:
@Composable
enjoyable Greeting(identify: String, modifier: Modifier = Modifier) {
Textual content(
textual content = "Howdy $identify!",
modifier = modifier
)
}
There are two issues of notice on this code. First, it’s a operate. Second, it’s annotated with @Composable. That is all it’s worthwhile to create a UI part in Compose, or, in Compose converse, a composable.
You would possibly’ve observed that the operate identify, Greeting(), is capitalized. Composable capabilities use Pascal case, not like the camel case generally utilized in Kotlin code. For instance, a multi-word Compose operate could be referred to as ConversationContent as a substitute of conversationContent. This distinction stems from composable capabilities returning UI objects, therefore adopting the identical naming conference as courses.
Greet Your self
Replace the worth of Greeting() from “Android” to your identify in order that Kodeco Chat says hi there to you.
You would possibly instantly see the worth change from “Android” to your identify on the gadget or emulator with out rerunning the app. In that case, that is due to a Compose and Android Studio function referred to as Stay Edit. When you don’t see it replace reside, run the app or allow Stay Edit in Android Studio’s settings.
Construct and run the app. When Android Studio finishes constructing and putting in, Kodeco Chat will seem in your gadget or emulator:
Exploring Actions
A way of life of assorted actions — like cardio, power coaching, and endurance — can hold you wholesome. Though they’re all totally different, they every have a particular function or aim.
Android apps are related — they’re constructed round a set of screens. Every display screen is called an Exercise and constructed round a single job. For instance, you might need a settings display screen the place customers can regulate the app’s settings or a sign-in display screen the place customers can log in with a username and password.
Within the Challenge navigator on the left, be sure that the app folder is expanded. Navigate to MainActivity.kt in app/kotlin+java/com.kodeco.chat/MainActivity.kt.
Open the file, and also you’ll see:
bundle com.kodeco.chat
import android.os.Bundle
import androidx.exercise.ComponentActivity
import androidx.exercise.compose.setContent
import androidx.compose.basis.format.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Floor
import androidx.compose.material3.Textual content
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.kodeco.chat.ui.theme.KodecoChatTheme
// 1
class MainActivity : ComponentActivity() {
// 2
override enjoyable onCreate(savedInstanceState: Bundle?) {
// 3
tremendous.onCreate(savedInstanceState)
// 4
setContent {
KodecoChatTheme {
// A floor container utilizing the 'background' shade from the theme
Floor(
modifier = Modifier.fillMaxSize(),
shade = MaterialTheme.colorScheme.background
) {
Greeting("Fuad")
}
}
}
}
}
@Composable
enjoyable Greeting(identify: String, modifier: Modifier = Modifier) {
Textual content(
textual content = "Howdy $identify!",
modifier = modifier
)
}
@Preview(showBackground = true)
@Composable
enjoyable GreetingPreview() {
KodecoChatTheme {
Greeting("Fuad")
}
}
MainActivity.kt is the place the logic in your chat display screen goes. Take a second to discover what it does:
- MainActivity is asserted as extending ComponentActivity. It’s your first and solely Exercise on this app. What ComponentActivity does isn’t necessary proper now. All it’s worthwhile to know is that you could subclass to take care of content material on the display screen.
- onCreate() is the entry level to this Exercise. It begins with the key phrase override, that means you’ll have to supply a customized implementation from the bottom ComponentActivity class.
- Calling the bottom’s implementation of onCreate() isn’t solely necessary — it’s required. You do that by calling tremendous.onCreate(). Android must arrange just a few issues itself earlier than your implementation executes, so that you notify the bottom class that it may possibly accomplish that now.
- This line “composes” the given composable — all the pieces that follows it within the braces {} — into the Exercise. The content material will turn out to be the basis view of the Exercise. The setContent{} block defines the Exercise’s format the place composable capabilities are referred to as. Composable capabilities can solely be referred to as from different composable capabilities. Therefore, you see the Greeting inside onCreate() is simply one other operate, outlined beneath that, however it’s additionally marked with @Composable, which makes it a composable operate.
Jetpack Compose makes use of a Kotlin compiler plugin to remodel these composable capabilities into the app’s UI components. For instance, the Textual content composable operate inside Greeting is, in flip, outlined by the Compose UI library and shows a textual content label on the display screen. You write composable capabilities to outline a view format that renders in your gadget display screen.
The place to Go From Right here?
Imagine it or not, you’re simply getting began with one of many 4 modules within the new Foundational Tools in Android course. By the tip of it, you’ll construct one thing that appears like this:
The course the next modules, which can take you from complete newbie to a spot the place you’re comfy with the basics of Android programming:
- Introduction to Model Management
- Introduction to Android Studio
- Meet the Kotlin Programming Language
- Create a Easy Android App
You possibly can expertise this new content material in certainly one of two methods:
- The Foundational Tools in Android course is accessible now, and free for all Kodeco Personal and Kodeco for Business subscribers.
- When you choose a holistic studying journey, guided by skilled mentors and culminating with a certificates of commencement, take a look at Kodeco’s brand-new Foundational Tools in Android on-demand bootcamp. You’ll be capable of be taught at your personal tempo, with the complete help of an skilled Android developer, as you navigate participating and difficult homework assignments that complement your studying of the topic supplies.
This course is designed for complete novices, and supplies a mild on-ramp for individuals keen to start out creating Android apps. Kodeco’s strategy to studying permits you to code alongside the professionals with demos, and homework for on-demand bootcamps.
If you wish to be taught Android, there’s no higher time!