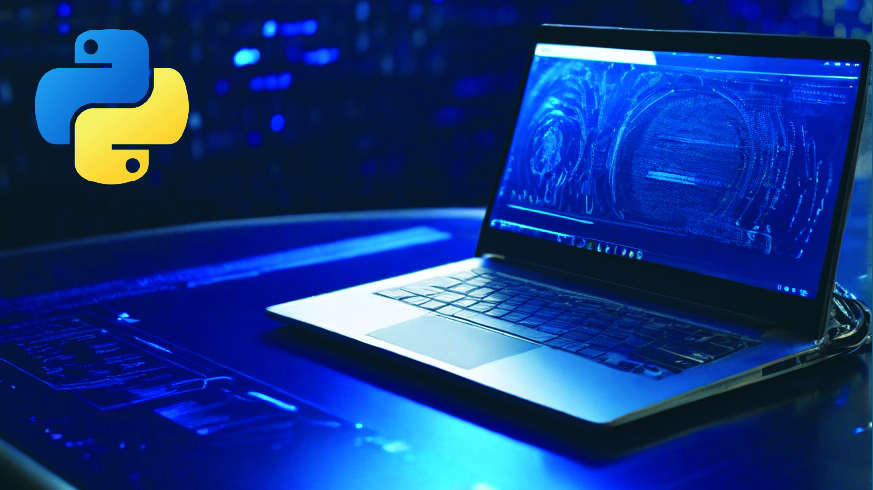
Introduction
String matching in Python might be difficult, however Pregex makes it straightforward with its easy and environment friendly pattern-matching capabilities. On this article, we are going to discover how Pregex may help you discover patterns in textual content effortlessly. We are going to cowl the advantages of utilizing Pregex, a step-by-step information to getting began, sensible examples, suggestions for environment friendly string matching, integration with different Python libraries, and finest practices to observe. Whether or not you’re a newbie or an skilled programmer, Pregex can simplify your string-matching duties and improve your Python initiatives.
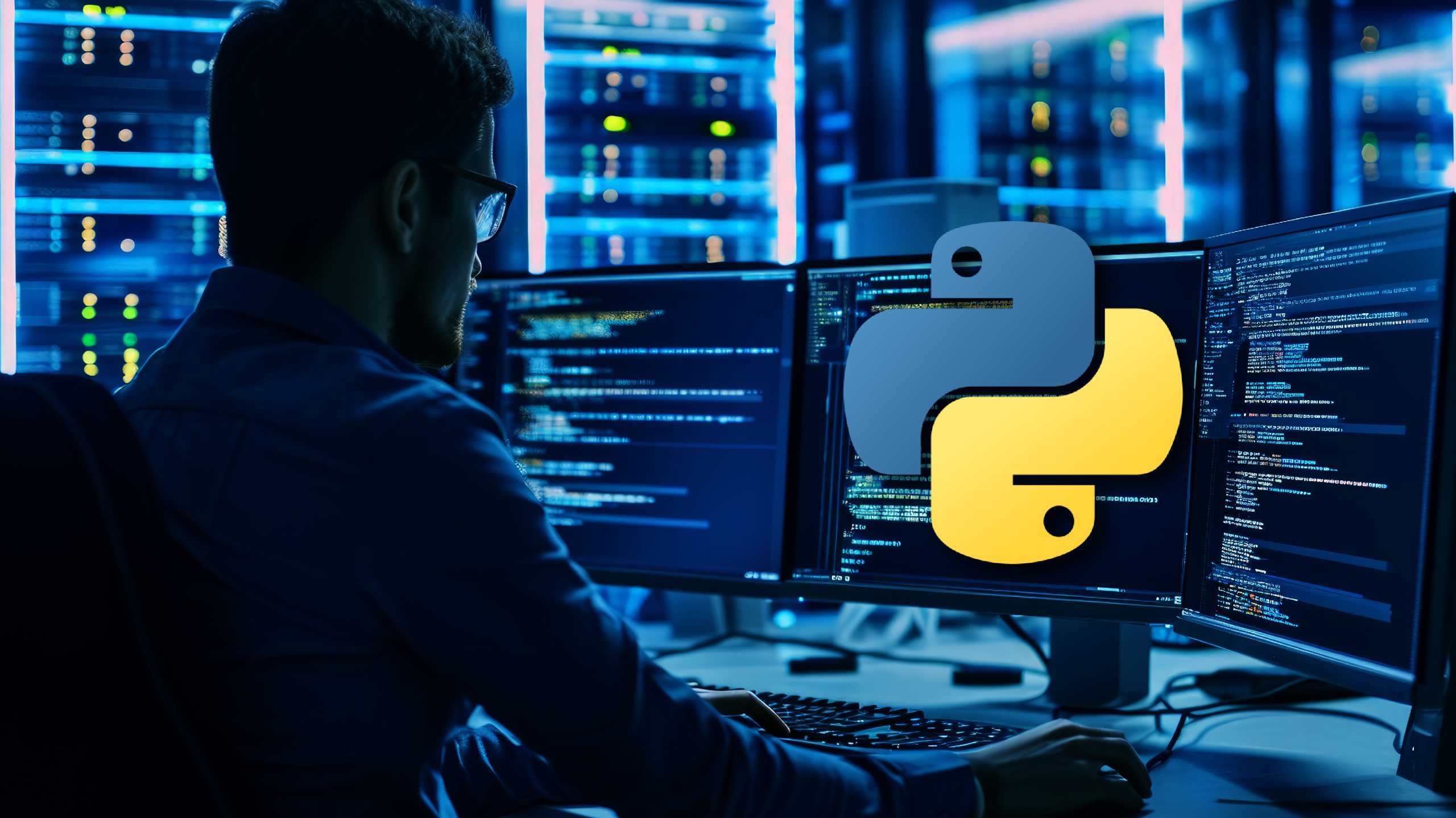
Advantages of Utilizing Pregex for String Matching
Pregex is a Python utility that simplifies the method of figuring out patterns in textual content with out requiring data of advanced programming. As a result of it simplifies and manages the code, Pregex advantages novice and seasoned programmers. Pregex makes organising and making use of patterns straightforward, accelerating growth and decreasing error charges. Moreover, this accessibility facilitates faster code updates and debugging, sustaining initiatives’ flexibility and effectivity.
Getting Began with Pregex in Python
You should first set up the library to begin utilizing Pregex in your Python project. You’ll be able to simply set up Pregex utilizing pip:
pip set up pregex
Fundamental Sample Matching
After you have put in Pregex, you should utilize it to do fundamental sample matching. For instance, to examine if a string accommodates a particular phrase, you should utilize the next code:
from pregex.core.pre import Pregex
textual content = "Howdy, World!"
sample = Pregex("Howdy")
end result = sample.get_matches(textual content)
if end result:
print("Sample discovered!")
else:
print("Sample not discovered.")
Output: Sample discovered!
Clarification
- Import the Pregex class from the pregex.core.pre module.
- Outline the textual content to go looking:
- textual content = “Howdy, World!”: That is the textual content during which we need to discover the sample.
- Create a sample:
- sample = Pregex(“Howdy”): This creates a Pregex object with the sample “Howdy”.
- Discover matches:
- end result = sample.get_matches(textual content): This makes use of the get_matches technique to seek out occurrences of the sample “Howdy” within the textual content.
- Examine and print outcomes:
- The if assertion checks if any matches had been discovered.
- If matches are discovered, it prints “Sample discovered!”.
- If no matches are discovered, it prints “Sample not discovered.”
Superior Sample Matching Methods
Pregex additionally helps superior pattern-matching strategies reminiscent of utilizing anchors, quantifiers, grouping, and capturing matches. These strategies help you create extra advanced patterns for matching strings.
Examples of String Matching with Pregex
Matching E mail Addresses
textual content="Howdy there, [email protected]"
from pregex.core.courses import AnyButFrom
from pregex.core.quantifiers import OneOrMore, AtLeast
from pregex.core.assertions import MatchAtLineEnd
person = OneOrMore(AnyButFrom("@", ' '))
firm = OneOrMore(AnyButFrom("@", ' ', '.'))
area = MatchAtLineEnd(AtLeast(AnyButFrom("@", ' ', '.'), 3))
pre = (
person +
"@" +
firm +
'.' +
area
)
outcomes = pre.get_matches(textual content)
print(outcomes)
Output: [‘[email protected]’]
Clarification
- Import needed Pregex courses:
- AnyButFrom: Matches any character besides these specified.
- OneOrMore: Matches a number of occurrences of the previous aspect.
- AtLeast: Matches not less than a specified variety of occurrences of the previous aspect.
- MatchAtLineEnd: Asserts that the next sample have to be on the finish of the road.
- Outline patterns for e-mail elements:
- person: Matches the half earlier than the “@” image (OneOrMore(AnyButFrom(“@”, ‘ ‘))).
- firm: Matches the half between the “@” image and the final dot (OneOrMore(AnyButFrom(“@”, ‘ ‘, ‘.’))).
- area: Matches the half after the final dot (MatchAtLineEnd(AtLeast(AnyButFrom(“@”, ‘ ‘, ‘.’), 3))).
- Mix the patterns:
- Concatenate person, “@”, firm, and area to type the entire e-mail sample.
- Discover matches within the textual content:
- Use the get_matches technique to seek out and print any e-mail addresses within the textual content.
Extracting URLs, Figuring out Telephone Numbers, and Parsing Knowledge from Textual content might be performed equally utilizing Pregex.
Additionally Learn: Introduction to Strings in Python For Beginners
Suggestions for Environment friendly String Matching with Pregex
Utilizing Anchors and Quantifiers, Grouping and Capturing Matches, Dealing with Particular Characters, and Efficiency Optimization are important for environment friendly string matching with Pregex.
Integrating Pregex with Different Python Libraries
Pregex might be seamlessly built-in with different Python libraries, reminiscent of Pandas, Common Expressions, and NLP libraries, to boost its performance and utility in numerous purposes.
Finest Practices for String Matching with Pregex
Writing clear and concise patterns, testing and validating patterns, and error dealing with and exception administration are a few of the finest practices to observe when working with Pregex for string matching.
Additionally Learn: String Data Structure in Python | Complete Case study
Conclusion
In conclusion, Pregex is a helpful instrument for string matching in Python, providing an easier and extra intuitive method than conventional common expressions. By following the ideas and finest practices outlined on this article, you possibly can leverage Pregex’s energy to match strings in your Python initiatives effectively. So, give Pregex a attempt to streamline your string-matching duties at present!
For extra articles on Python, discover our article section at present.